-
What makes up the Model, View and Controller in Struts 2?
- Controller: FilterDispatcher
- Model: Action
- View: Result
-
What is a Controller?
The controller's job is to map requests to actions.
-
What is a FilterDispatcher? What does it do?
- FilterDispatcher is a servlet filter.
- It forms the Controller in the MVC pattern.
- It inspects each incoming request to determine which Struts 2 action should handle the request.
- Maps URL to Action class.
- FilterDispatcher is deprecated (>= v2.1.3) instead use StrutsPrepareAndExecuteFilter.
-
What is a Model?
- The model is the internal state of the application.
- It consists of the Data model and the business logic.
-
What is an Action? What does it do?
- Action is an encapsulation of calls to the business logic in a single unit of work.
- Action also serves as the single point of data transfer.
-
What is a View?
It is the presentation component of the MVC framework/pattern.
-
What is a Result? What does it do?
- Result form the View component of the MVC pattern.
- It passes on the state (result of a request) of the application to the client using JSP,Velocity, FreeMarker, and XSLT.
-
Who decides which Result needs to be called?
Action
-
Describe the request processing work-flow in Struts 2.
-
What are Interceptors?
- Interceptors are stack of classes.
- The call to the Action passes through the Interceptors.
- The Interceptors are also invoked after the Result has executed.
- Interceptors need not do something both times.
-
What are common examples of Interceptors?
- Logging
- Data Validation
- Type Conversion
- File Upload
-
What is a ValueStack?
A central storage area, that holds all the data associated with the processing of a request.
-
What is OGNL?
OGNL is the tool that allows us to access the data from the ValueStack.
-
Who all can access the ValueStack?
The ValueStack is available to all components involved in the processing of a request -
- Interceptors
- Actions
- Results
-
How is ValueStack available through out the request processing flow?
ValueStack is stored in a ThreadLocal context called ActionContext.
-
What is an ActionContext?
- ActionContext contains all the data that makes up the context for an Action (request).
- It is made up of ValueStack, request object, session object, application maps, etc.
-
What is the significance of ThreadLocal object in the context of ActionContext and/or ValueStack?
The use of ThreadLocal makes the ActionContext, and thus the ValueStack, accessible from anywhere in the same thread of execution.
-
FilterDispatcher is now deprecated. What filter replaces it?
StrutsPrepareAndExecuteFilter
-
Where do you specify to Struts 2, the place where it can find the annotations? (in case we are using annotations instead of xml)
- We specify that in the web.xml
- As init-param to the filter declaration.
- <filter>
- <filter-name>struts2</filter-name>
- <filter-class>
- org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter
- </filter-class>
- <init-param>
- <param-name>
- actionPackages
- </param-name>
- <param-value>
- com.mycompany.myapp.actions
- </param-value>
- </init-param>
- </filter>
-
Can there be multiple struts.xml files? How would you use them?
Yes. By 'including' them in the central struts.xml file.
- <struts>
- <include file="me/rishabh/anotherStruts.xml"/>
- </struts>
-
Is it necessary to implement the Action interface?
No. But your action class should have the execute method that returns a String object, directing the flow onwards.
- public String execute() {
- return "SUCCESS";
- }
-
How can you set configuration properties for Struts?
- By using the constant tag in struts.xml.
- By creating a struts.properties file.
-
What is Struts 2 Namespace configuration?
- Used to handle multiple modules, using a namespace.
- Also allows for same name actions, across different namespaces.
-
How does Struts scan for annotations? Elaborate on the "Scanning Methodology" and "Naming Converter" mechanism.
- I. Scanning Methodology:
- 1. Look for Action classes in the folder declared as <init-param>actionPackages</init-param>
- 2. Look for action classes in folders named 'action', 'actions', 'struts' and 'struts2'.
- 3. Scan for files that match either of the following:
- a. Implemented the com.opensymphony.xwork2.Action interface.
- b. Extends the com.opensymphony.xwork2.ActionSupport class.
- c. File name ends with Action (e.g UserAction, LoginAction).
- II. Naming Converter:
- 1. Drop the ending 'Action' from class name, if any.
- 2. Convert the first letter of the file name to lowercase.
- E.g.: The class named LoginAction, will translate to action name - login OR login.action
-
Is Action class in Struts 2 multithreaded? Is it singleton?
- Action class is not multithreaded.
- Action class is not singleton.
- Struts create a new instance of Action class for every request.
-
Can your package reuse another namespace?
Can you use the same namespace across packages?
Yes.
-
What are the attributes of the Struts 2 package?
- name (required): Name of the package.
- namespace: Namespace for all actions in package.
- extends: Parent package to inherit from.
- abstract: If 'true', this package will only be used to define inheritable components, not actions.
-
What happens when you don't set the namespace attribute for a package?
The actions will go to the 'default' namespace, i.e. the blank namespace - "".
-
Talk about the 'struts-default' package
- It is defined in the struts-default.xml file in the core jar.
- Declares commonly used components like interceptor stack and result types.
-
How are the parameters from the form copied over to the member variables of the Action class and vice-versa?
With the help of 'param' interceptor (ParametersInterceptor) available under the 'struts-default' package.
-
What is ActionSupport class? What is it used for?
- The ActionSupport class implements the Action interface.
- Validation: Declared a validate() method and put the validation code inside.
- Text localization: Use GetText() method to get the message from resource bundle.
- It is highly recommended to use ActionSupport class everytime, unless you have a reason to not use it.
-
What are the different mechanism of data transfer between ValueStack and the Action and/or JSPs
- Object-backed JavaBeans properties
- ModelDriven actions
-
Talk about Object-backed JavaBeans properties for data transfer.
- Instead of using individual properties in the Action class, we can use an Object.
- We need not instantiate the object. The framework does it for us.
- Ensure that the JSPs refer the properties through the object name. E.g.- user.username
-
What is ModelDriven? Elaborate.
- It is an interface.
- Action class needs to implement it.
- Has only one method - public Object getModel()
- The object need to be created/instantiated explicitly by us at the class level.
- Ensure that you do not modify or create a new reference for the model, as the framework would continue to point to the old one. (the one created at class level)
- Code:
- public class SomeAction implements ModelDriven {
- private User user = new User();
- public Object getModel() { return user; }
- }
-
How to remove/replace the .action suffix/extension from URLs?
- Add the following constant to the struts.xml file
- <constant name="struts.action.extension" value=""/>
-
Uploading files in Struts 2
- JSP: Use multipart form. Use post method.
- <s:form method="post" action="fileUpload" namespace="/Upload" enctype="multipart/form-data">
- <p>Upload File Here</p>
- <s:file name="pic" />
- <s:submit />
- </s:form>
- Action: Need a File property with name same as declared in the JSP. And supporting props too.
- File pic;
- String picContentType;
- String picFileName;
- Struts.xml: Extend the struts-default package
-
What does ActionInvocation do?
- It encapsulates the execution of an Action, along with its interceptors and the Result.
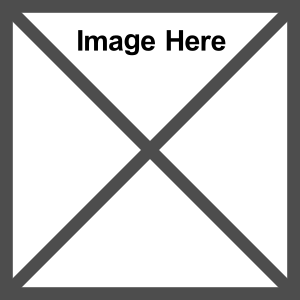
-
What is the order of invocation of interceptors?
- In the order declared in the struts.xml.
- After result, the interceptors are called in reversed order.
- Interceptor-1 -> Interceptor-N -> Action -> Result -> Interceptor-N -> Interceptor-1
-
How does ActionInvocation work?
- ActionInvocation exposes invoke() method for the framework.
- Then it in turns call the intercept() methods on the Interceptors.
- The intercept() method itself takes an ActionInvocation object. So that the invoke() can be called to pass onto the next interceptor.
-
How to create your own Interceptors?
- Implement the Interceptor interface.
- Define the method
- public String intercept(ActionInvocation invocation) throws Exception {
- // pre-processing code here
- String result = invocation.invoke();
- // post-processing code here
- return result;
- }
-
How to declare Interceptors?
- <package name="cust-intercepts">
- <interceptors>
- <interceptor name="logger" class="LogInterceptor"/>
- ...
-
- <interceptor-stack name="customStack">
- <interceptor-ref name="exception"/>
- </interceptor-stack>
- </interceptors>
- </package>
|
|